Divyanshu loves to spend his time drawing, sketching and painting.…
Python is a universal and accessible programming language. It is also useful for developing applications that work on different platforms. Thanks to frameworks like Kivy, Python allows you to create cross-platform applications for different devices and operating systems.
Table of Contents
ToggleWhy Python Is the Finest Choice for Software Development
In addition to being accsessible, Python is also a popular language with open source code and a powerful community. Developers prefer it for its simplicity and wide functions.
Compared to languages like C++, Kotlin, Swift, and JavaScript, Python offers:
- Clear and rich syntax. Python’s syntax is fairly easy to understand, which lowers the barrier to entry and the learning curve. It is easy to apply and modify.
- Large libraries. A large number of open-source packages are available to optimize development, which is an important support in the process of creating complex products.
- Active support of the community. As already mentioned, an active community is always ready to help solve problems and share knowledge. Python has such a community that is a significant factor forcing its active development.
- Versatile applications. You can use Python for web development, data analysis, artificial intelligence, machine learning, and many more things. This versatility makes it a valuable language for various projects and industries.
- Extensive documentation. Python’s extensive documentation and a wealth of tutorials, guides, and forums. This is what makes it easier for developers to learn, troubleshoot, and improve their coding skills.
Additionally, frameworks like BeeWare and Kivy allow developers to use a single code base to build across multiple platforms. In other words, it eliminates the need to develop separate apps for iOS and Android.
Kivy for Multi-Platform App Development
Kivy is a Python library that makes it easy to build cross-platform applications. It provides a graphical user interface that makes the development process interactive, fast, and convenient. Before diving into coding, it is very important to define the problem that your application will solve.
One of the main features of Kivy worth special attention is its ability to handle multi-touch events. This capability is particularly useful for developing mobile applications with an emphasis on touch interactions, accordingly. Using Kivy’s multi-touch support, developers can create more responsive and intuitive user interfaces.
In general, apart from developing mobile solutions, Kivy’s support for various input devices makes it a versatile tool for developing applications that must run on different types of hardware, from smartphones to tablets and desktops.
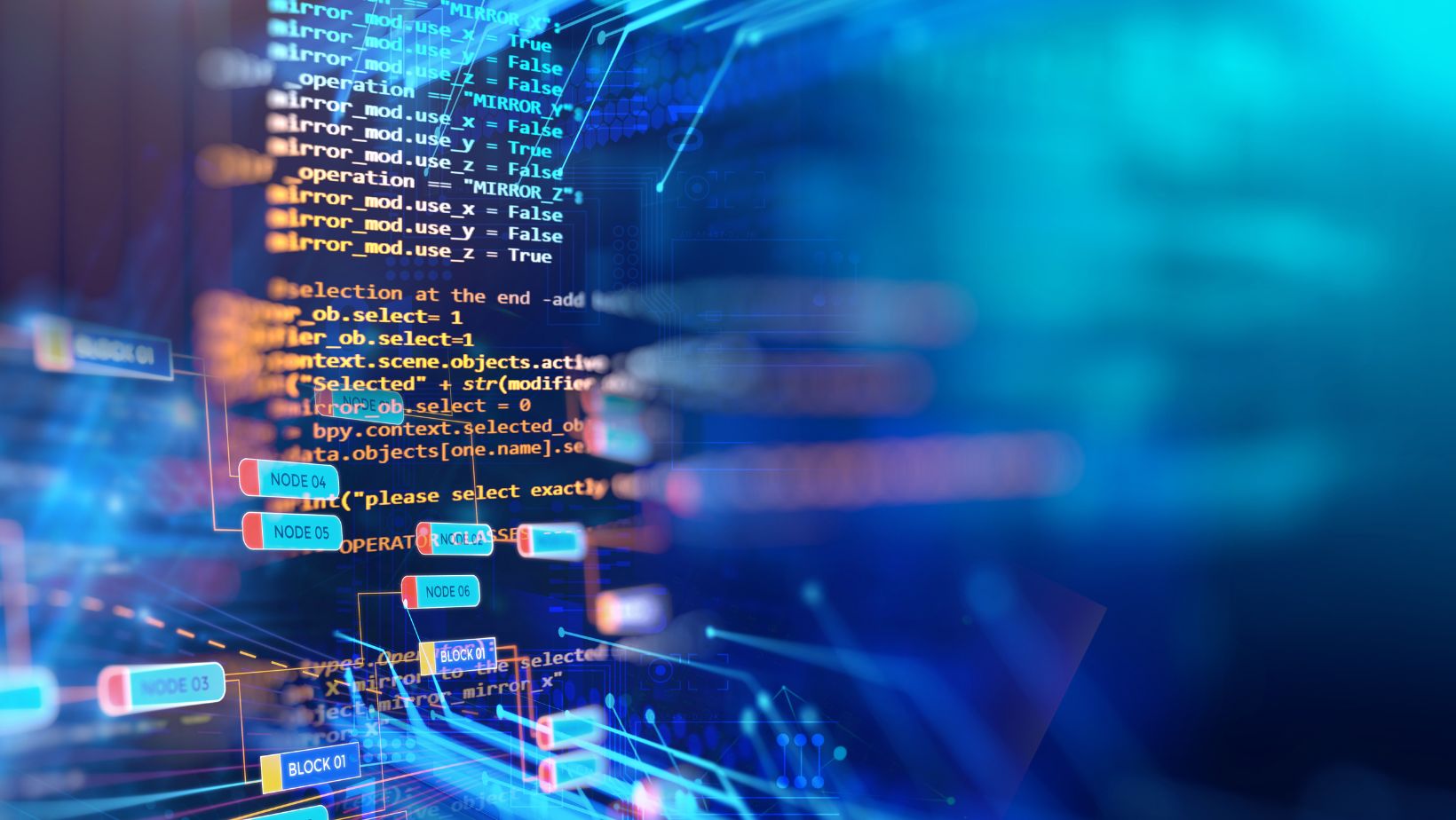
Another benefit of using Kivy is its rich ecosystem of widgets and tools that simplify the development process. This framework includes a wide set of ready-made widgets, such as buttons, labels, sliders, and text input. You can easily customize it to the specific needs of your application.
This not only speeds up and simplifies development, but also ensures a consistent look and feel across platforms. In addition, the framework’s flexible architecture allows for easy integration with other Python libraries. This way you can extend the functionality of your applications without having to reinvent the wheel.
In this guide, we will create an Age Calculator app that will help users quickly determine their age. We’ll explore how to use Kivy’s labels, buttons, text inputs, and images to create a user-friendly interface and briefly discuss the practical advantages of Python software development services.
Getting Started with Kivy
Assuming you are familiar with Python programming and its objects and classes. Let’s get started.
First, install the Kivy library with the following command:
pip install kivy
Once installed, import the Kivy library. To do this, you should create a folder named “calculator_app” and within it, a file called main.py.
This file is going to be the main script for your app.
Step 1: Setting Up Application Widgets
Start by importing the necessary Kivy widgets and initializing the main application class, AgeCalculator. This class will create the window object for the app.
from kivy.app import App
from kivy.uix.gridlayout import GridLayout
from kivy.uix.label import Label
from kivy.uix.button import Button
from kivy.uix.textinput import TextInput
class AgeCalculator(App):
def build(self):
self.window = GridLayout()
self.window.cols = 1
# Add widgets here
# …
return self.window
if __name__ == “__main__”:
AgeCalculator().run()
Step 2: Creating User Input Fields
Next, we have to add a label and text input so user can enter their year of birth.
self.window.add_widget(Label(text=”Enter your Year of Birth:”))
self.year_input = TextInput(multiline=False)
self.window.add_widget(self.year_input)
Step 3: Adding a Button and Binding a Function
Now, we can add a button that will trigger the age calculation when pressed. Bind this button to a callback function.
self.calculate_button = Button(text=”Calculate Age”)
self.calculate_button.bind(on_press=self.calculate_age)
self.window.add_widget(self.calculate_button)
Step 4: The Age Calculation Function
Here, we can define the calculate_age function to compute the user’s age based on the current year and the input provided.
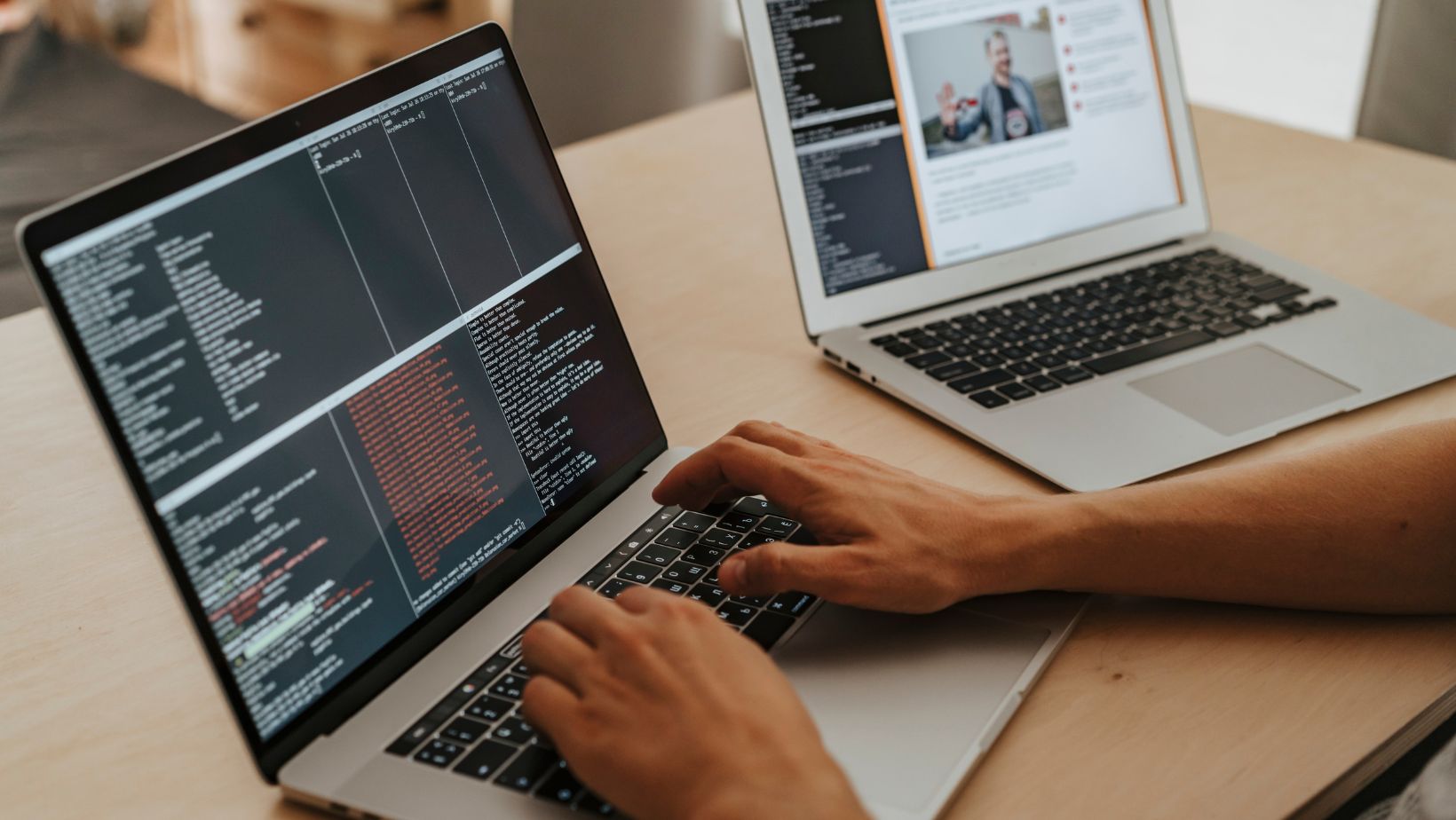
from datetime import datetime
def calculate_age(self, instance):
current_year = datetime.now().year
birth_year = int(self.year_input.text)
age = current_year – birth_year
self.window.add_widget(Label(text=f”Your age is: {age}”))
Step 5: Styling the App’s User Interface
Looks nice, doesn’t it? Now, we can enhance the app’s appearance by adjusting margins and layout settings.
self.window.padding = [10, 10, 10, 10]
self.window.spacing = 10
Step 6: Running the Application
Easy-peasy! To run the app, just navigate to the project directory in your terminal. Here, you need to execute the following command:
python main.py
Wrapping Up
As you can see, building an application in Python, especially using the Kivy framework, opens up a whole world of possibilities for developing cross-platform applications with a single codebase. You can easily set up development environments, design user interfaces, and implement core features.
Python’s clear and rich syntax, extensive libraries, and active community support make it the best choice for many purposes and developers with different approaches and philosophies. Kivy Framework, in turn, simplifies the creation of cross-platform applications. It offers a robust graphical user interface and simplifies the development process. Kivy’s ability to run on multiple operating systems without modifying the codebase significantly reduces development team time and effort.
With Python and Kivy, you can get a lot of possibilities. We have only shown a simple example of using these technologies, but with more extensive knowledge, you can use them to create a variety of applications such as games, productivity tools, and more.
Divyanshu loves to spend his time drawing, sketching and painting. He also enjoys writing blogs on various topics that interest him. He is a witty and intelligent person, who likes to engage in interesting conversations with people he meets. He is someone you would love to know!